“Has anyone figured out how to remove a default alignment on a core block? For example, Media & Text defaults to wide and I don’t want that.” Yesterday, Melissa asked this question in one of the Slacks we’re both in.
Yes! You definitely can with a Block Filter! You can, in fact, filter and manipulate all the basic settings of a block with a filter called blocks.registerBlockType
.
Skip right to the solution, or keep on reading for a thorough understanding.
About blocks.registerBlockType
The blocks.registerBlockType
provides you with:
- an object of all the block settings, and
- the name of the block being registered.
Just like regular WordPress filters, the first parameter is what you manipulate, and any other parameters provided are to help you do what you need to do.
We can manipulate the settings object – remove, add, or modify any of the settings and pass that back to the block registration. Depending on what you want to do, the easiest way to manipulate the object is by using a lodash
function. lodash
is a utility library which gives us access to the kinds of functions and methods we are used to in PHP.
But how do you find out what settings a block has?
- Look at the source code (typically the
block.json
file) of the block ,or - Run the filter with a
console.log
command in your code like so:
/**
* WordPress dependencies
*/
import { addFilter } from "@wordpress/hooks";
/**
* Call the filter
*
* Filter name: blocks.registerBlockType
* Unique identifier for our change: aurooba-makes-look-at-settings
* The nameless function that manipulates the passed data is the third parameter
*/
addFilter(
"blocks.registerBlockType",
"aurooba-makes-look-at-settings",
(settings, name) => {
console.log(settings);
return settings;
}
);
You can save that code in a .js file and enqueue like shown in this code block.
This will show you the setting object for every block you have registered on your website, in the browser console:
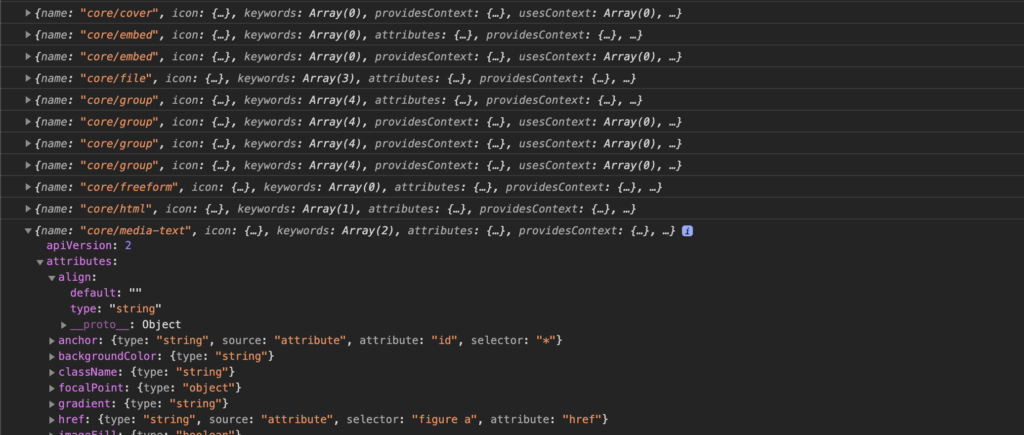
So to answer Melissa’s question, we’re going to use the lodash.merge()
function, which takes in an object and the modifications and merges it together, replacing the original parts we modified, and leaving the rest of the object intact. (Here is a longer explanation of how lodash.merge()
works.)
First, we’re going to target only the Media & Text block, by checking the name of the block. Then we’ll create an object that mimics the hierarchy of the settings object and provides a different default value for the align attribute.
Next we’ll use lodash.merge()
to modify the settings object with our new default value, then pass it to the block registration.
Let’s see that in code:
Here is the complete (and heavily documented) JavaScript code you would enqueue:
/**
* WordPress dependencies
*/
import { addFilter } from "@wordpress/hooks";
/**
* Change the alignment default for the Media & Text block
*
* @param {object} settings
* @param {string} name
*/
function removeDefaultAlignment(settings, name) {
// if the current block is not the Media & Text block, move on
if (name !== "core/media-text") {
return settings;
}
/**
* We create a variable that assigns the setting we want to change, maintaining the levels in the original
* settings object. The original settings object is as follows:
*
* settings: {
* attributes: {
* type: "string",
* "default": "wide"
* }
* othersettings..
* }
*
* */
let changeDefaultAttribute = {
attributes: {
align: {
default: "",
},
},
};
/**
* We are merging the original settings and our new setting, which replaces only
* the setting we have a new value for, and then putting it in a
* new mergedSettings object
*
* {} : the empty object which will be stored in mergedSettings
* settings : the original settings object
* changeDefaultAttribute : our modified setting object
*/
let mergedSettings = lodash.merge({}, settings, changeDefaultAttribute);
// send back the mergedSettings
return mergedSettings;
}
/**
* Call the filter
*
* Filter name: blocks.registerBlockType
* Unique identifier for our change: aurooba-makes-remove-media-text-default-align
* The function that manipulated the passed data: removeDefaultAlignment
*/
addFilter(
"blocks.registerBlockType",
"aurooba-makes-remove-media-text-default-align",
removeDefaultAlignment
);
To enqueue this into the Block Editor, you would add the following code to your plugin or functions.php
file:
/**
* Enqueue script into the Block Editor
*
* @return void
*/
function aurooba_makes_block_modifications() {
$index_js = 'build/index.js';
// Enqueue block editor JS
wp_enqueue_script(
'my-block-editor-js',
plugins_url( $index_js, __FILE__ ),
array( 'wp-blocks', 'wp-hooks' ),
filemtime( plugin_dir_path( __FILE__ ) . $index_js ),
true
);
}
// Hook the enqueue functions into the editor
add_action( 'enqueue_block_editor_assets', 'aurooba_makes_block_modifications' );
Now if you add the Media & Text block, it won’t be set to the wide alignment by default, because we’ve emptied the default
parameter.
As long as you maintain the correct hierarchy when modifying a setting, the lodash.merge
function will take care of it for you.
If you found this helpful, you’ll enjoy my Block Editor Essentials email series, where I show you the helpful modifications you can make to the Block Editor experience (and blocks!). There’s so much more to the Block Editor than just custom blocks.