It’s fairly simple to add a custom colour palette to the Block Editor (Gutenberg). It’s simple enough that my designer friends can do it without any help from a developer, as long as they have access to the code block they’ll need to drop into the functions.php
file.
This is a modified excerpt from my free Block Editor Essentials series that teaches you how to develop with the Block Editor.
There are two parts to adding a custom colour palette:
- The PHP – including the new palette so it shows up in the Block Editor, and enqueuing the CSS styles into the Block Editor for an accurate editing experience.
- The CSS – adding the appropriate classes that will create the desired colour effects in both the Block Editor and on the front-end.
The PHP
IMPORTANT: Add all your custom PHP to the very END of your functions.php file.
The first function you’ll add will include the custom colour palette in the Block Editor settings:
function aa_custom_block_editor_palette () {
add_theme_support(
'editor-color-palette',
[
[
'name' => esc_html__( 'COLOUR', 'auroobamakes' ),
'slug' => 'colour',
'color' => 'HEXCODE',
],
[
'name' => esc_html__( 'COLOUR', 'auroobamakes' ),
'slug' => 'colouir',
'color' => 'HEXCODE',
],
[
'name' => esc_html__( 'COLOUR NAME', 'auroobamakes' ),
'slug' => 'colour-name',
'color' => '#c6d8d3',
],
]
);
}
add_action( 'after_setup_theme', 'aa_custom_block_editor_palette' );
This example is set up to add 3 custom colours, but you can add as many as you like. You’ll copy paste lines 15-19 right underneath line 19 to add a new colour.
There are 3 parts to each colour:
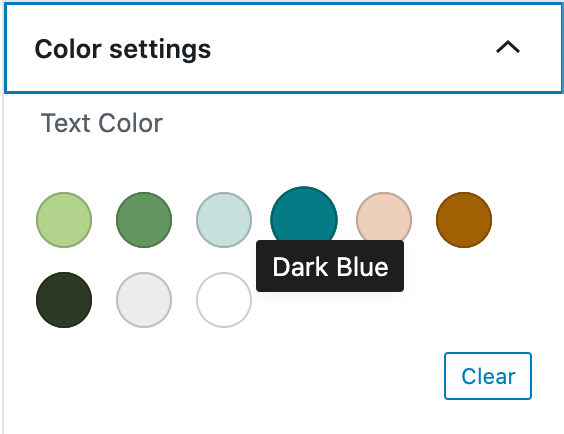
- Name – when someone hovers on the colour in the palette, this is what will appear. This name is translation ready and can include spaces, symbols, whatever you like.
- Slug – the slug has all the rules a permalink slug does (keep it lowercase, no spaces, use dashes). It’ll be used in the CSS class associated with this colour.
- Hex code – this is the hex code of the colour you want to add.
The second function you’ll add will enqueue a stylesheet into your Block Editor so the preview includes the colour changes as you select colours in your new palette:
/**
*
* Enqueue stylesheet to Block Editor as well
* (good for child themes where you're just adding the palette CSS)
* Otherwise enqueue a different sheet for the Block Editor
*
*/
function aa_add_block_editor_stylesheet() {
// Add support for editor styles.
add_theme_support( 'editor-styles' );
// Enqueue editor styles.
add_editor_style( 'style.css' );
}
add_action( 'after_setup_theme', 'aa_add_block_editor_stylesheet' );
This function first declares support for editor-styles
and then enqueues the editor stylesheet. In this case, I’ve just added the theme stylesheet, style.css
, which works well for child themes where the stylesheet is being used JUST to add custom Block Editor styles.
However, if you are not using style.css in that way, you should create a new CSS file called editor.css
(or any other name you like), add it to the root of your theme (where style.css also lives) and replace the style.css
in line 13 with that filename.
The CSS
When you add custom colours to the Block Editor palette and use them, one of two classes are applied to the block:
.has-COLOUR-background-color
or
.has-COLOUR-color
So you need to create these classes in your CSS file (in style.css
and in editor.css
) and include the hex code for the colour:
/* add these two classes for EVERY colour you add */
.has-COLOUR-background-color {
background-color: HEXCODE;
}
.has-COLOUR-color {
color: HEXCODE;
}
Once you do this, your colour palette will function correctly everywhere you use it. 🙂
Bonus: SCSS for faster class creation!
The idea of having to create two new classes for every colour I use makes me want to throw my keyboard at something.
However, with SCSS (Sass), this process becomes much simpler. We create a map (aka list/array) that will have the slugs as the key and the hex code as the value, and then loop over the map to create the new classes:
// SASS VERSION
// Add slugs as the name of each colour and then the hex code
$palette: (
colour: #FF0000,
colour-two: #001EFF,
colour-three: #00FF00,
colour-four: #F6FF00,
);
// no need to touch this part, this will create all the necessary classes
@each $colour in $palette {
.has-#{nth($colour, 1)}-background-color {
background: nth($colour, 2);
}
.has-#{nth($colour, 1)}-color {
color:nth($colour, 2);
}
}
There you have it. 🙂